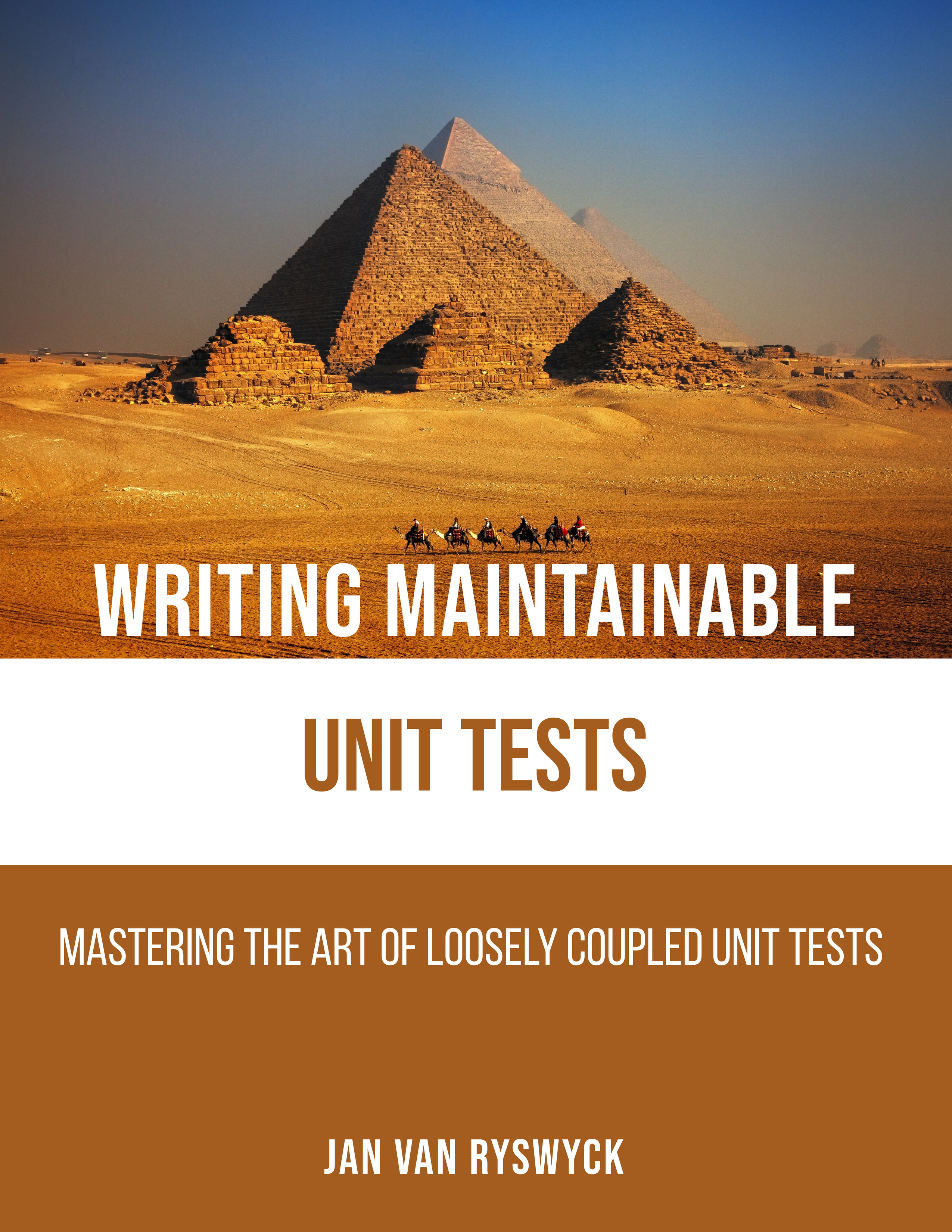
Be careful with optional parameters in C# 4.0
November 9, 2008I've been playing with the Visual Studio 2010 CTP bits, and I tried to see what named and optional parameters in C# 4.0 can bring to the table. Although they are minor language enhancements at best, they can cause a lot of grief as well.
In my former life as a C++ developer, optional parameters were quite handy in some cases to remove some ceremonial code for providing obvious overloads of a method. Since I came over to the .NET platform, I actually don't miss optional parameters. I just wanted to share a common abuse of optional parameters that you should be aware of. The following code illustrates this:
public class Base
{
public virtual void Foo(Int32 x = 1)
{}
}
public class Derived
{
public override void Foo(Int32 x = 2)
{}
}
...
Base f0 = new Base();
f0.Foo(); // Invokes Foo(1)
Base f1 = new Derived();
f1.Foo(); // Also invokes Foo(1)
Derived f2 = new Derived();
f2.Foo(); // Invokes Foo(2)
This is something you have to watch out for. The object's member function silently takes different arguments depending on the static type you use. This violates the principle of least surprise and is a general PITA. Don't violate the method contract that has been provided by the base class.
Don't say I didn't warn you ;-)
If you and your team want to learn more about how to write maintainable unit tests and get the most out of TDD practices, make sure to have look at our trainings and workshops or check out the books section. Feel free to reach out at info. @ principal-it .be
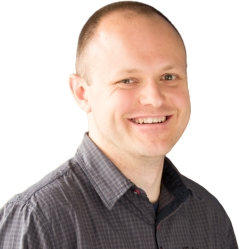
Jan Van Ryswyck
Thank you for visiting my blog. I’m a professional software developer since Y2K. A blogger since Y2K+5. Provider of training and coaching in XP practices. Curator of the Awesome Talks list. Past organizer of the European Virtual ALT.NET meetings. Thinking and learning about all kinds of technologies since forever.
Comments
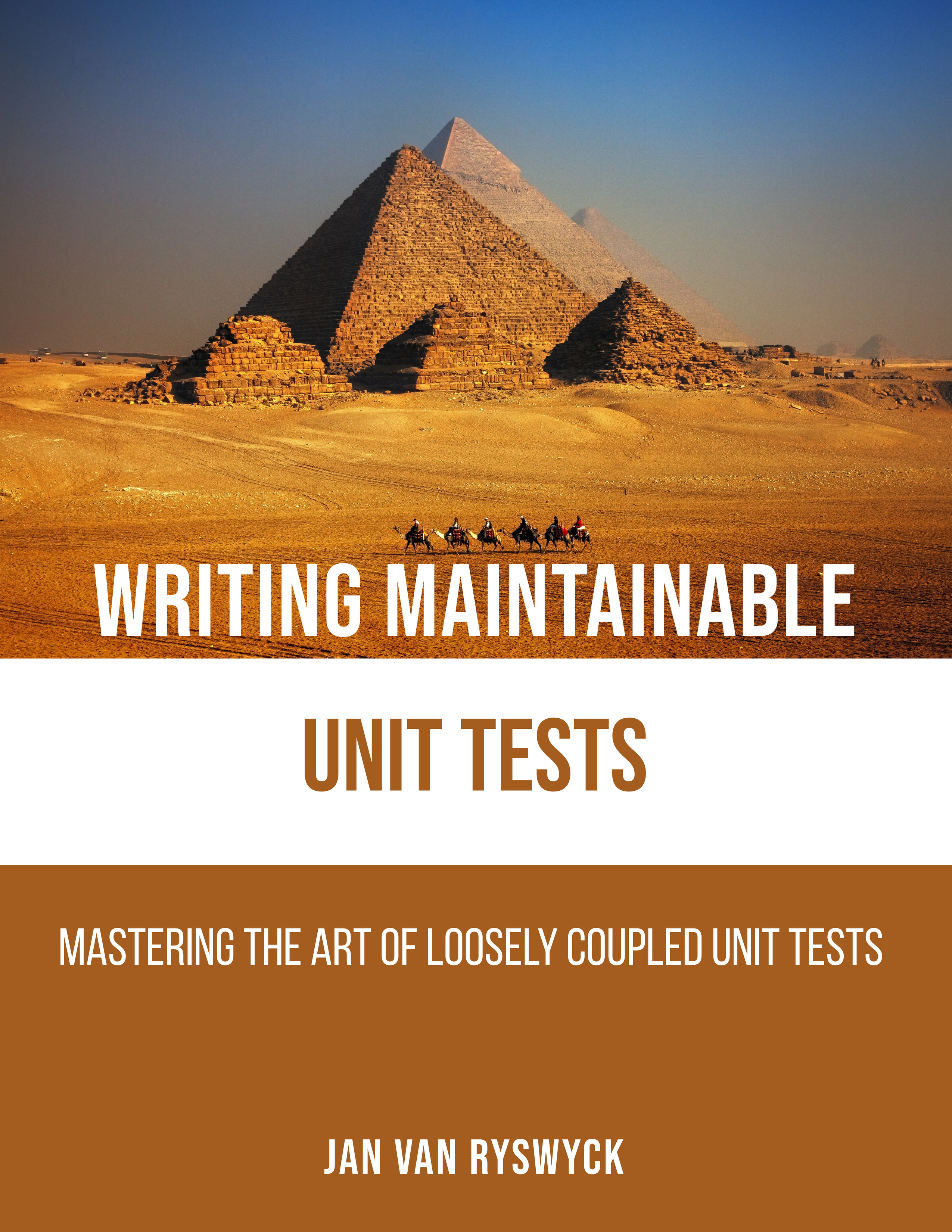
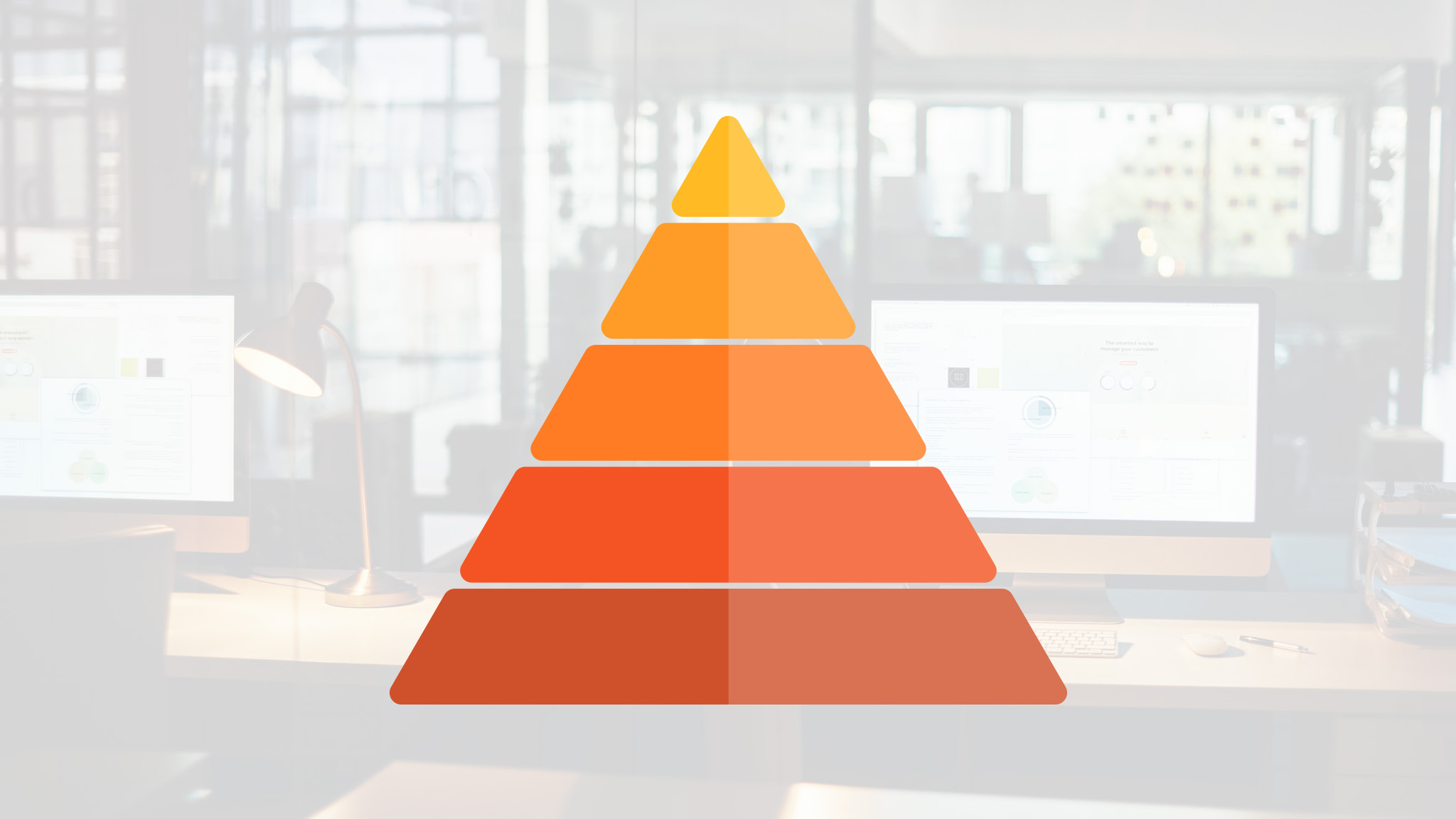
Writing Maintainable
Unit Tests
Watch The Videos
Latest articles
-
Contract Tests - Parameterised Test Cases
June 28, 2023
-
Contract Tests - Abstract Test Cases
April 12, 2023
-
Contract Tests
February 1, 2023
-
The Testing Quadrant
June 15, 2022
-
Tales Of TDD: The Big Refactoring
February 2, 2022
Tags
- .NET
- ALT.NET
- ASP.NET
- Agile
- Announcement
- Architecture
- Behavior-Driven Development
- C++
- CQRS
- Clojure
- CoffeeScript
- Community
- Concurrent Programming
- Conferences
- Continuous Integration
- Core Skills
- CouchDB
- Database
- Design Patterns
- Domain-Driven Design
- Event Sourcing
- F#
- Fluent Interfaces
- Functional Programming
- Hacking
- Humor
- Java
- JavaScript
- Linux
- Microsoft
- NHibernate
- NoSQL
- Node.js
- Object-Relational Mapping
- Open Source
- Reading
- Ruby
- Software Design
- SourceControl
- Test-Driven Development
- Testing
- Tools
- Visual Studio
- Web
- Windows
Disclaimer
The opinions expressed on this blog are my own personal opinions. These do NOT represent anyone else’s view on the world in any way whatsoever.
About
Thank you for visiting my website. I’m a professional software developer since Y2K. A blogger since Y2K+5. Author of Writing Maintainable Unit Tests. Provider of training and coaching in XP practices. Curator of the Awesome Talks list. Thinking and learning about all kinds of technologies since forever.
Latest articles
Contract Tests - Parameterised Test Cases
Contract Tests - Abstract Test Cases
Contract Tests
The Testing Quadrant
Contact information
(+32) 496 38 00 82
info @ principal-it .be